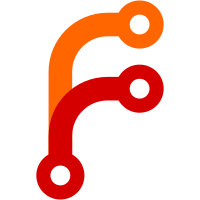
This is the OSS portion of enterprise PR 1994 Rather than directly interrogating the agent-local state for HTTP checks using the `HTTPCheckFetcher` interface, we now rely on the config snapshot containing the checks. This reduces the number of changes required to support server xDS sessions. It's not clear why the fetching approach was introduced in 931d167ebb2300839b218d08871f22323c60175d.
481 lines
13 KiB
Go
481 lines
13 KiB
Go
package xds
|
|
|
|
import (
|
|
"path/filepath"
|
|
"sort"
|
|
"testing"
|
|
"time"
|
|
|
|
envoy_route_v3 "github.com/envoyproxy/go-control-plane/envoy/config/route/v3"
|
|
"google.golang.org/protobuf/types/known/durationpb"
|
|
|
|
testinf "github.com/mitchellh/go-testing-interface"
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"github.com/hashicorp/consul/agent/proxycfg"
|
|
"github.com/hashicorp/consul/agent/structs"
|
|
"github.com/hashicorp/consul/agent/xds/proxysupport"
|
|
"github.com/hashicorp/consul/agent/xds/xdscommon"
|
|
"github.com/hashicorp/consul/sdk/testutil"
|
|
)
|
|
|
|
func TestRoutesFromSnapshot(t *testing.T) {
|
|
|
|
tests := []struct {
|
|
name string
|
|
create func(t testinf.T) *proxycfg.ConfigSnapshot
|
|
overrideGoldenName string
|
|
}{
|
|
{
|
|
name: "defaults",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshot(t, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "connect-proxy-with-chain",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "simple", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "connect-proxy-with-chain-external-sni",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "external-sni", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "connect-proxy-with-chain-and-overrides",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "simple-with-overrides", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "splitter-with-resolver-redirect",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "splitter-with-resolver-redirect-multidc", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "connect-proxy-with-chain-and-splitter",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "chain-and-splitter", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "connect-proxy-with-grpc-router",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "grpc-router", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "connect-proxy-with-chain-and-router",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "chain-and-router", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "connect-proxy-lb-in-resolver",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotDiscoveryChain(t, "lb-resolver", nil, nil)
|
|
},
|
|
},
|
|
// TODO(rb): test match stanza skipped for grpc
|
|
// Start ingress gateway test cases
|
|
{
|
|
name: "ingress-defaults-no-chain",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, false, "tcp",
|
|
"default", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-chain",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, true, "tcp",
|
|
"simple", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-chain-external-sni",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, true, "tcp",
|
|
"external-sni", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-splitter-with-resolver-redirect",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, true, "http",
|
|
"splitter-with-resolver-redirect-multidc", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-chain-and-splitter",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, true, "http",
|
|
"chain-and-splitter", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-grpc-router",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, true, "http",
|
|
"grpc-router", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-chain-and-router",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, true, "http",
|
|
"chain-and-router", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-lb-in-resolver",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGateway(t, true, "http",
|
|
"lb-resolver", nil, nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-http-multiple-services",
|
|
create: proxycfg.TestConfigSnapshotIngress_HTTPMultipleServices,
|
|
},
|
|
{
|
|
name: "ingress-grpc-multiple-services",
|
|
create: proxycfg.TestConfigSnapshotIngress_GRPCMultipleServices,
|
|
},
|
|
{
|
|
name: "ingress-with-chain-and-router-header-manip",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGatewayWithChain(t, "router-header-manip", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-sds-listener-level",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGatewayWithChain(t, "sds-listener-level", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-sds-listener-level-wildcard",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGatewayWithChain(t, "sds-listener-level-wildcard", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-sds-service-level",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGatewayWithChain(t, "sds-service-level", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "ingress-with-sds-service-level-mixed-tls",
|
|
create: func(t testinf.T) *proxycfg.ConfigSnapshot {
|
|
return proxycfg.TestConfigSnapshotIngressGatewayWithChain(t, "sds-service-level-mixed-tls", nil, nil)
|
|
},
|
|
},
|
|
{
|
|
name: "terminating-gateway-lb-config",
|
|
create: proxycfg.TestConfigSnapshotTerminatingGatewayLBConfig,
|
|
},
|
|
}
|
|
|
|
latestEnvoyVersion := proxysupport.EnvoyVersions[0]
|
|
for _, envoyVersion := range proxysupport.EnvoyVersions {
|
|
sf, err := determineSupportedProxyFeaturesFromString(envoyVersion)
|
|
require.NoError(t, err)
|
|
t.Run("envoy-"+envoyVersion, func(t *testing.T) {
|
|
for _, tt := range tests {
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
// Sanity check default with no overrides first
|
|
snap := tt.create(t)
|
|
|
|
// We need to replace the TLS certs with deterministic ones to make golden
|
|
// files workable. Note we don't update these otherwise they'd change
|
|
// golden files for every test case and so not be any use!
|
|
setupTLSRootsAndLeaf(t, snap)
|
|
|
|
g := newResourceGenerator(testutil.Logger(t), nil, false)
|
|
g.ProxyFeatures = sf
|
|
|
|
routes, err := g.routesFromSnapshot(snap)
|
|
require.NoError(t, err)
|
|
|
|
sort.Slice(routes, func(i, j int) bool {
|
|
return routes[i].(*envoy_route_v3.RouteConfiguration).Name < routes[j].(*envoy_route_v3.RouteConfiguration).Name
|
|
})
|
|
r, err := createResponse(xdscommon.RouteType, "00000001", "00000001", routes)
|
|
require.NoError(t, err)
|
|
|
|
t.Run("current", func(t *testing.T) {
|
|
gotJSON := protoToJSON(t, r)
|
|
|
|
gName := tt.name
|
|
if tt.overrideGoldenName != "" {
|
|
gName = tt.overrideGoldenName
|
|
}
|
|
|
|
require.JSONEq(t, goldenEnvoy(t, filepath.Join("routes", gName), envoyVersion, latestEnvoyVersion, gotJSON), gotJSON)
|
|
})
|
|
})
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestEnvoyLBConfig_InjectToRouteAction(t *testing.T) {
|
|
var tests = []struct {
|
|
name string
|
|
lb *structs.LoadBalancer
|
|
expected *envoy_route_v3.RouteAction
|
|
}{
|
|
{
|
|
name: "empty",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: "",
|
|
},
|
|
// we only modify route actions for hash-based LB policies
|
|
expected: &envoy_route_v3.RouteAction{},
|
|
},
|
|
{
|
|
name: "least request",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: structs.LBPolicyLeastRequest,
|
|
LeastRequestConfig: &structs.LeastRequestConfig{
|
|
ChoiceCount: 3,
|
|
},
|
|
},
|
|
// we only modify route actions for hash-based LB policies
|
|
expected: &envoy_route_v3.RouteAction{},
|
|
},
|
|
{
|
|
name: "headers",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: "ring_hash",
|
|
RingHashConfig: &structs.RingHashConfig{
|
|
MinimumRingSize: 3,
|
|
MaximumRingSize: 7,
|
|
},
|
|
HashPolicies: []structs.HashPolicy{
|
|
{
|
|
Field: structs.HashPolicyHeader,
|
|
FieldValue: "x-route-key",
|
|
Terminal: true,
|
|
},
|
|
},
|
|
},
|
|
expected: &envoy_route_v3.RouteAction{
|
|
HashPolicy: []*envoy_route_v3.RouteAction_HashPolicy{
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Header_{
|
|
Header: &envoy_route_v3.RouteAction_HashPolicy_Header{
|
|
HeaderName: "x-route-key",
|
|
},
|
|
},
|
|
Terminal: true,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "cookies",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: structs.LBPolicyMaglev,
|
|
HashPolicies: []structs.HashPolicy{
|
|
{
|
|
Field: structs.HashPolicyCookie,
|
|
FieldValue: "red-velvet",
|
|
Terminal: true,
|
|
},
|
|
{
|
|
Field: structs.HashPolicyCookie,
|
|
FieldValue: "oatmeal",
|
|
},
|
|
},
|
|
},
|
|
expected: &envoy_route_v3.RouteAction{
|
|
HashPolicy: []*envoy_route_v3.RouteAction_HashPolicy{
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Cookie_{
|
|
Cookie: &envoy_route_v3.RouteAction_HashPolicy_Cookie{
|
|
Name: "red-velvet",
|
|
},
|
|
},
|
|
Terminal: true,
|
|
},
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Cookie_{
|
|
Cookie: &envoy_route_v3.RouteAction_HashPolicy_Cookie{
|
|
Name: "oatmeal",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "non-zero session ttl gets zeroed out",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: structs.LBPolicyMaglev,
|
|
HashPolicies: []structs.HashPolicy{
|
|
{
|
|
Field: structs.HashPolicyCookie,
|
|
FieldValue: "oatmeal",
|
|
CookieConfig: &structs.CookieConfig{
|
|
TTL: 10 * time.Second,
|
|
Session: true,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
expected: &envoy_route_v3.RouteAction{
|
|
HashPolicy: []*envoy_route_v3.RouteAction_HashPolicy{
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Cookie_{
|
|
Cookie: &envoy_route_v3.RouteAction_HashPolicy_Cookie{
|
|
Name: "oatmeal",
|
|
Ttl: durationpb.New(0 * time.Second),
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "zero value ttl omitted if not session cookie",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: structs.LBPolicyMaglev,
|
|
HashPolicies: []structs.HashPolicy{
|
|
{
|
|
Field: structs.HashPolicyCookie,
|
|
FieldValue: "oatmeal",
|
|
CookieConfig: &structs.CookieConfig{
|
|
Path: "/oven",
|
|
},
|
|
},
|
|
},
|
|
},
|
|
expected: &envoy_route_v3.RouteAction{
|
|
HashPolicy: []*envoy_route_v3.RouteAction_HashPolicy{
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Cookie_{
|
|
Cookie: &envoy_route_v3.RouteAction_HashPolicy_Cookie{
|
|
Name: "oatmeal",
|
|
Path: "/oven",
|
|
Ttl: nil,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "source addr",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: structs.LBPolicyMaglev,
|
|
HashPolicies: []structs.HashPolicy{
|
|
{
|
|
SourceIP: true,
|
|
Terminal: true,
|
|
},
|
|
},
|
|
},
|
|
expected: &envoy_route_v3.RouteAction{
|
|
HashPolicy: []*envoy_route_v3.RouteAction_HashPolicy{
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_ConnectionProperties_{
|
|
ConnectionProperties: &envoy_route_v3.RouteAction_HashPolicy_ConnectionProperties{
|
|
SourceIp: true,
|
|
},
|
|
},
|
|
Terminal: true,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
{
|
|
name: "kitchen sink",
|
|
lb: &structs.LoadBalancer{
|
|
Policy: structs.LBPolicyMaglev,
|
|
HashPolicies: []structs.HashPolicy{
|
|
{
|
|
SourceIP: true,
|
|
Terminal: true,
|
|
},
|
|
{
|
|
Field: structs.HashPolicyCookie,
|
|
FieldValue: "oatmeal",
|
|
CookieConfig: &structs.CookieConfig{
|
|
TTL: 10 * time.Second,
|
|
Path: "/oven",
|
|
},
|
|
},
|
|
{
|
|
Field: structs.HashPolicyCookie,
|
|
FieldValue: "chocolate-chip",
|
|
CookieConfig: &structs.CookieConfig{
|
|
Session: true,
|
|
Path: "/oven",
|
|
},
|
|
},
|
|
{
|
|
Field: structs.HashPolicyHeader,
|
|
FieldValue: "special-header",
|
|
Terminal: true,
|
|
},
|
|
},
|
|
},
|
|
expected: &envoy_route_v3.RouteAction{
|
|
HashPolicy: []*envoy_route_v3.RouteAction_HashPolicy{
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_ConnectionProperties_{
|
|
ConnectionProperties: &envoy_route_v3.RouteAction_HashPolicy_ConnectionProperties{
|
|
SourceIp: true,
|
|
},
|
|
},
|
|
Terminal: true,
|
|
},
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Cookie_{
|
|
Cookie: &envoy_route_v3.RouteAction_HashPolicy_Cookie{
|
|
Name: "oatmeal",
|
|
Ttl: durationpb.New(10 * time.Second),
|
|
Path: "/oven",
|
|
},
|
|
},
|
|
},
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Cookie_{
|
|
Cookie: &envoy_route_v3.RouteAction_HashPolicy_Cookie{
|
|
Name: "chocolate-chip",
|
|
Ttl: durationpb.New(0 * time.Second),
|
|
Path: "/oven",
|
|
},
|
|
},
|
|
},
|
|
{
|
|
PolicySpecifier: &envoy_route_v3.RouteAction_HashPolicy_Header_{
|
|
Header: &envoy_route_v3.RouteAction_HashPolicy_Header{
|
|
HeaderName: "special-header",
|
|
},
|
|
},
|
|
Terminal: true,
|
|
},
|
|
},
|
|
},
|
|
},
|
|
}
|
|
|
|
for _, tc := range tests {
|
|
t.Run(tc.name, func(t *testing.T) {
|
|
var ra envoy_route_v3.RouteAction
|
|
err := injectLBToRouteAction(tc.lb, &ra)
|
|
require.NoError(t, err)
|
|
|
|
require.Equal(t, tc.expected, &ra)
|
|
})
|
|
}
|
|
}
|