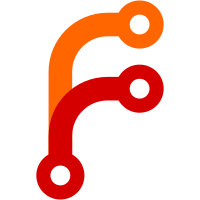
These changes are necessary to ensure advertisement happens correctly even when datacenters are connected via network areas in Consul enterprise. This also changes how we check if ACLs can be upgraded within the local datacenter. Previously we would iterate through all LAN members. Now we just use the ServerLookup type to iterate through all known servers in the DC.
71 lines
1.5 KiB
Go
71 lines
1.5 KiB
Go
// +build !consulent
|
|
|
|
package consul
|
|
|
|
import (
|
|
"net"
|
|
|
|
"github.com/hashicorp/consul/agent/pool"
|
|
"github.com/hashicorp/consul/agent/structs"
|
|
"github.com/hashicorp/go-version"
|
|
"github.com/hashicorp/serf/serf"
|
|
)
|
|
|
|
var (
|
|
// minMultiDCConnectVersion is the minimum version in order to support multi-DC Connect
|
|
// features.
|
|
minMultiDCConnectVersion = version.Must(version.NewVersion("1.6.0"))
|
|
)
|
|
|
|
type EnterpriseServer struct{}
|
|
|
|
func (s *Server) initEnterprise() error {
|
|
return nil
|
|
}
|
|
|
|
func (s *Server) startEnterprise() error {
|
|
return nil
|
|
}
|
|
|
|
func (s *Server) handleEnterpriseUserEvents(event serf.UserEvent) bool {
|
|
return false
|
|
}
|
|
|
|
func (s *Server) handleEnterpriseRPCConn(rtype pool.RPCType, conn net.Conn, isTLS bool) bool {
|
|
return false
|
|
}
|
|
|
|
func (s *Server) handleEnterpriseNativeTLSConn(alpnProto string, conn net.Conn) bool {
|
|
return false
|
|
}
|
|
|
|
func (s *Server) handleEnterpriseLeave() {
|
|
return
|
|
}
|
|
|
|
func (s *Server) enterpriseStats() map[string]map[string]string {
|
|
return nil
|
|
}
|
|
|
|
func (s *Server) establishEnterpriseLeadership() error {
|
|
return nil
|
|
}
|
|
|
|
func (s *Server) revokeEnterpriseLeadership() error {
|
|
return nil
|
|
}
|
|
|
|
func (s *Server) validateEnterpriseRequest(entMeta *structs.EnterpriseMeta, write bool) error {
|
|
return nil
|
|
}
|
|
|
|
func (_ *Server) addEnterpriseSerfTags(_ map[string]string) {
|
|
// do nothing
|
|
}
|
|
|
|
// updateEnterpriseSerfTags in enterprise will update any instances of Serf with the tag that
|
|
// are not the normal LAN or WAN serf instances (network segments and network areas)
|
|
func (_ *Server) updateEnterpriseSerfTags(_, _ string) {
|
|
// do nothing
|
|
}
|