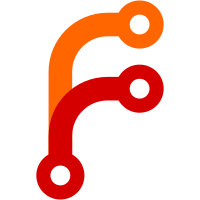
This brings down the test run from 108 sec to 15 sec. There is an occasional port conflict because of the nature the next port is chosen. So far it seems rare enough to live with it.
36 lines
611 B
Go
36 lines
611 B
Go
package agent
|
|
|
|
import (
|
|
"flag"
|
|
"reflect"
|
|
"testing"
|
|
)
|
|
|
|
func TestAppendSliceValue_implements(t *testing.T) {
|
|
t.Parallel()
|
|
var raw interface{}
|
|
raw = new(AppendSliceValue)
|
|
if _, ok := raw.(flag.Value); !ok {
|
|
t.Fatalf("AppendSliceValue should be a Value")
|
|
}
|
|
}
|
|
|
|
func TestAppendSliceValueSet(t *testing.T) {
|
|
t.Parallel()
|
|
sv := new(AppendSliceValue)
|
|
err := sv.Set("foo")
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
err = sv.Set("bar")
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
expected := []string{"foo", "bar"}
|
|
if !reflect.DeepEqual([]string(*sv), expected) {
|
|
t.Fatalf("Bad: %#v", sv)
|
|
}
|
|
}
|