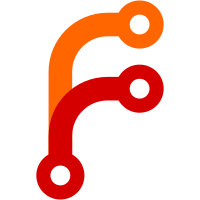
TLDR with many modules the versions included in each diverged quite a bit. Attempting to use Go Workspaces produces a bunch of errors. This commit: 1. Fixes envoy-library-references.sh to work again 2. Ensures we are pulling in go-control-plane@v0.11.0 everywhere (previously it was at that version in some modules and others were much older) 3. Remove one usage of golang/protobuf that caused us to have a direct dependency on it. 4. Remove deprecated usage of the Endpoint field in the grpc resolver.Target struct. The current version of grpc (v1.55.0) has removed that field and recommended replacement with URL.Opaque and calls to the Endpoint() func when needing to consume the previous field. 4. `go work init <all the paths to go.mod files>` && `go work sync`. This syncrhonized versions of dependencies from the main workspace/root module to all submodules 5. Updated .gitignore to ignore the go.work and go.work.sum files. This seems to be standard practice at the moment. 6. Update doc comments in protoc-gen-consul-rate-limit to be go fmt compatible 7. Upgraded makefile infra to perform linting, testing and go mod tidy on all modules in a flexible manner. 8. Updated linter rules to prevent usage of golang/protobuf 9. Updated a leader peering test to account for an extra colon in a grpc error message.
1051 lines
38 KiB
Go
1051 lines
38 KiB
Go
// Copyright (c) HashiCorp, Inc.
|
|
// SPDX-License-Identifier: MPL-2.0
|
|
|
|
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.30.0
|
|
// protoc (unknown)
|
|
// source: pbcatalog/v1alpha1/health.proto
|
|
|
|
package catalogv1alpha1
|
|
|
|
import (
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
durationpb "google.golang.org/protobuf/types/known/durationpb"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type Health int32
|
|
|
|
const (
|
|
// buf:lint:ignore ENUM_ZERO_VALUE_SUFFIX
|
|
Health_HEALTH_ANY Health = 0
|
|
Health_HEALTH_PASSING Health = 1
|
|
Health_HEALTH_WARNING Health = 2
|
|
Health_HEALTH_CRITICAL Health = 3
|
|
Health_HEALTH_MAINTENANCE Health = 4
|
|
)
|
|
|
|
// Enum value maps for Health.
|
|
var (
|
|
Health_name = map[int32]string{
|
|
0: "HEALTH_ANY",
|
|
1: "HEALTH_PASSING",
|
|
2: "HEALTH_WARNING",
|
|
3: "HEALTH_CRITICAL",
|
|
4: "HEALTH_MAINTENANCE",
|
|
}
|
|
Health_value = map[string]int32{
|
|
"HEALTH_ANY": 0,
|
|
"HEALTH_PASSING": 1,
|
|
"HEALTH_WARNING": 2,
|
|
"HEALTH_CRITICAL": 3,
|
|
"HEALTH_MAINTENANCE": 4,
|
|
}
|
|
)
|
|
|
|
func (x Health) Enum() *Health {
|
|
p := new(Health)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x Health) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (Health) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_pbcatalog_v1alpha1_health_proto_enumTypes[0].Descriptor()
|
|
}
|
|
|
|
func (Health) Type() protoreflect.EnumType {
|
|
return &file_pbcatalog_v1alpha1_health_proto_enumTypes[0]
|
|
}
|
|
|
|
func (x Health) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use Health.Descriptor instead.
|
|
func (Health) EnumDescriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
// This resource will belong to a workload or a node and will have an ownership relationship.
|
|
type HealthStatus struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// Type is the type of this health check, such as http, tcp, or kubernetes-readiness
|
|
Type string `protobuf:"bytes,1,opt,name=type,proto3" json:"type,omitempty"`
|
|
// Health is the status. This maps to existing health check statuses.
|
|
Status Health `protobuf:"varint,2,opt,name=status,proto3,enum=hashicorp.consul.catalog.v1alpha1.Health" json:"status,omitempty"`
|
|
// Description is the description for this status.
|
|
Description string `protobuf:"bytes,3,opt,name=description,proto3" json:"description,omitempty"`
|
|
// Output is the output from running the check that resulted in this status
|
|
Output string `protobuf:"bytes,4,opt,name=output,proto3" json:"output,omitempty"`
|
|
}
|
|
|
|
func (x *HealthStatus) Reset() {
|
|
*x = HealthStatus{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *HealthStatus) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*HealthStatus) ProtoMessage() {}
|
|
|
|
func (x *HealthStatus) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use HealthStatus.ProtoReflect.Descriptor instead.
|
|
func (*HealthStatus) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *HealthStatus) GetType() string {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HealthStatus) GetStatus() Health {
|
|
if x != nil {
|
|
return x.Status
|
|
}
|
|
return Health_HEALTH_ANY
|
|
}
|
|
|
|
func (x *HealthStatus) GetDescription() string {
|
|
if x != nil {
|
|
return x.Description
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HealthStatus) GetOutput() string {
|
|
if x != nil {
|
|
return x.Output
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type HealthChecks struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Workloads *WorkloadSelector `protobuf:"bytes,1,opt,name=workloads,proto3" json:"workloads,omitempty"`
|
|
HealthChecks []*HealthCheck `protobuf:"bytes,2,rep,name=health_checks,json=healthChecks,proto3" json:"health_checks,omitempty"`
|
|
}
|
|
|
|
func (x *HealthChecks) Reset() {
|
|
*x = HealthChecks{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *HealthChecks) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*HealthChecks) ProtoMessage() {}
|
|
|
|
func (x *HealthChecks) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use HealthChecks.ProtoReflect.Descriptor instead.
|
|
func (*HealthChecks) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *HealthChecks) GetWorkloads() *WorkloadSelector {
|
|
if x != nil {
|
|
return x.Workloads
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthChecks) GetHealthChecks() []*HealthCheck {
|
|
if x != nil {
|
|
return x.HealthChecks
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type HealthCheck struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Name string `protobuf:"bytes,1,opt,name=name,proto3" json:"name,omitempty"`
|
|
// Types that are assignable to Definition:
|
|
//
|
|
// *HealthCheck_Http
|
|
// *HealthCheck_Tcp
|
|
// *HealthCheck_Udp
|
|
// *HealthCheck_Grpc
|
|
// *HealthCheck_OsService
|
|
Definition isHealthCheck_Definition `protobuf_oneof:"definition"`
|
|
Interval *durationpb.Duration `protobuf:"bytes,7,opt,name=interval,proto3" json:"interval,omitempty"`
|
|
Timeout *durationpb.Duration `protobuf:"bytes,8,opt,name=timeout,proto3" json:"timeout,omitempty"`
|
|
DeregisterCriticalAfter *durationpb.Duration `protobuf:"bytes,9,opt,name=deregister_critical_after,json=deregisterCriticalAfter,proto3" json:"deregister_critical_after,omitempty"`
|
|
}
|
|
|
|
func (x *HealthCheck) Reset() {
|
|
*x = HealthCheck{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *HealthCheck) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*HealthCheck) ProtoMessage() {}
|
|
|
|
func (x *HealthCheck) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[2]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use HealthCheck.ProtoReflect.Descriptor instead.
|
|
func (*HealthCheck) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *HealthCheck) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *HealthCheck) GetDefinition() isHealthCheck_Definition {
|
|
if m != nil {
|
|
return m.Definition
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetHttp() *HTTPCheck {
|
|
if x, ok := x.GetDefinition().(*HealthCheck_Http); ok {
|
|
return x.Http
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetTcp() *TCPCheck {
|
|
if x, ok := x.GetDefinition().(*HealthCheck_Tcp); ok {
|
|
return x.Tcp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetUdp() *UDPCheck {
|
|
if x, ok := x.GetDefinition().(*HealthCheck_Udp); ok {
|
|
return x.Udp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetGrpc() *GRPCCheck {
|
|
if x, ok := x.GetDefinition().(*HealthCheck_Grpc); ok {
|
|
return x.Grpc
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetOsService() *OSServiceCheck {
|
|
if x, ok := x.GetDefinition().(*HealthCheck_OsService); ok {
|
|
return x.OsService
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetInterval() *durationpb.Duration {
|
|
if x != nil {
|
|
return x.Interval
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetTimeout() *durationpb.Duration {
|
|
if x != nil {
|
|
return x.Timeout
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HealthCheck) GetDeregisterCriticalAfter() *durationpb.Duration {
|
|
if x != nil {
|
|
return x.DeregisterCriticalAfter
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type isHealthCheck_Definition interface {
|
|
isHealthCheck_Definition()
|
|
}
|
|
|
|
type HealthCheck_Http struct {
|
|
Http *HTTPCheck `protobuf:"bytes,2,opt,name=http,proto3,oneof"`
|
|
}
|
|
|
|
type HealthCheck_Tcp struct {
|
|
Tcp *TCPCheck `protobuf:"bytes,3,opt,name=tcp,proto3,oneof"`
|
|
}
|
|
|
|
type HealthCheck_Udp struct {
|
|
Udp *UDPCheck `protobuf:"bytes,4,opt,name=udp,proto3,oneof"`
|
|
}
|
|
|
|
type HealthCheck_Grpc struct {
|
|
Grpc *GRPCCheck `protobuf:"bytes,5,opt,name=grpc,proto3,oneof"`
|
|
}
|
|
|
|
type HealthCheck_OsService struct {
|
|
OsService *OSServiceCheck `protobuf:"bytes,6,opt,name=os_service,json=osService,proto3,oneof"`
|
|
}
|
|
|
|
func (*HealthCheck_Http) isHealthCheck_Definition() {}
|
|
|
|
func (*HealthCheck_Tcp) isHealthCheck_Definition() {}
|
|
|
|
func (*HealthCheck_Udp) isHealthCheck_Definition() {}
|
|
|
|
func (*HealthCheck_Grpc) isHealthCheck_Definition() {}
|
|
|
|
func (*HealthCheck_OsService) isHealthCheck_Definition() {}
|
|
|
|
type HTTPCheck struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Url string `protobuf:"bytes,1,opt,name=url,proto3" json:"url,omitempty"`
|
|
Header map[string]string `protobuf:"bytes,2,rep,name=header,proto3" json:"header,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Method string `protobuf:"bytes,3,opt,name=method,proto3" json:"method,omitempty"`
|
|
Body string `protobuf:"bytes,4,opt,name=body,proto3" json:"body,omitempty"`
|
|
DisableRedirects bool `protobuf:"varint,5,opt,name=disable_redirects,json=disableRedirects,proto3" json:"disable_redirects,omitempty"`
|
|
Tls *CheckTLSConfig `protobuf:"bytes,6,opt,name=tls,proto3" json:"tls,omitempty"`
|
|
}
|
|
|
|
func (x *HTTPCheck) Reset() {
|
|
*x = HTTPCheck{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *HTTPCheck) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*HTTPCheck) ProtoMessage() {}
|
|
|
|
func (x *HTTPCheck) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[3]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use HTTPCheck.ProtoReflect.Descriptor instead.
|
|
func (*HTTPCheck) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *HTTPCheck) GetUrl() string {
|
|
if x != nil {
|
|
return x.Url
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HTTPCheck) GetHeader() map[string]string {
|
|
if x != nil {
|
|
return x.Header
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *HTTPCheck) GetMethod() string {
|
|
if x != nil {
|
|
return x.Method
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HTTPCheck) GetBody() string {
|
|
if x != nil {
|
|
return x.Body
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *HTTPCheck) GetDisableRedirects() bool {
|
|
if x != nil {
|
|
return x.DisableRedirects
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *HTTPCheck) GetTls() *CheckTLSConfig {
|
|
if x != nil {
|
|
return x.Tls
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type TCPCheck struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
}
|
|
|
|
func (x *TCPCheck) Reset() {
|
|
*x = TCPCheck{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *TCPCheck) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TCPCheck) ProtoMessage() {}
|
|
|
|
func (x *TCPCheck) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[4]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TCPCheck.ProtoReflect.Descriptor instead.
|
|
func (*TCPCheck) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
func (x *TCPCheck) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UDPCheck struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
}
|
|
|
|
func (x *UDPCheck) Reset() {
|
|
*x = UDPCheck{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UDPCheck) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UDPCheck) ProtoMessage() {}
|
|
|
|
func (x *UDPCheck) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[5]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UDPCheck.ProtoReflect.Descriptor instead.
|
|
func (*UDPCheck) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *UDPCheck) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type GRPCCheck struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
Tls *CheckTLSConfig `protobuf:"bytes,2,opt,name=tls,proto3" json:"tls,omitempty"`
|
|
}
|
|
|
|
func (x *GRPCCheck) Reset() {
|
|
*x = GRPCCheck{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *GRPCCheck) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*GRPCCheck) ProtoMessage() {}
|
|
|
|
func (x *GRPCCheck) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[6]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use GRPCCheck.ProtoReflect.Descriptor instead.
|
|
func (*GRPCCheck) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *GRPCCheck) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *GRPCCheck) GetTls() *CheckTLSConfig {
|
|
if x != nil {
|
|
return x.Tls
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type OSServiceCheck struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Address string `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
}
|
|
|
|
func (x *OSServiceCheck) Reset() {
|
|
*x = OSServiceCheck{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *OSServiceCheck) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*OSServiceCheck) ProtoMessage() {}
|
|
|
|
func (x *OSServiceCheck) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[7]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use OSServiceCheck.ProtoReflect.Descriptor instead.
|
|
func (*OSServiceCheck) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *OSServiceCheck) GetAddress() string {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type CheckTLSConfig struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
TlsServerName string `protobuf:"bytes,1,opt,name=tls_server_name,json=tlsServerName,proto3" json:"tls_server_name,omitempty"`
|
|
TlsSkipVerify bool `protobuf:"varint,2,opt,name=tls_skip_verify,json=tlsSkipVerify,proto3" json:"tls_skip_verify,omitempty"`
|
|
UseTls bool `protobuf:"varint,3,opt,name=use_tls,json=useTls,proto3" json:"use_tls,omitempty"`
|
|
}
|
|
|
|
func (x *CheckTLSConfig) Reset() {
|
|
*x = CheckTLSConfig{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *CheckTLSConfig) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*CheckTLSConfig) ProtoMessage() {}
|
|
|
|
func (x *CheckTLSConfig) ProtoReflect() protoreflect.Message {
|
|
mi := &file_pbcatalog_v1alpha1_health_proto_msgTypes[8]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use CheckTLSConfig.ProtoReflect.Descriptor instead.
|
|
func (*CheckTLSConfig) Descriptor() ([]byte, []int) {
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *CheckTLSConfig) GetTlsServerName() string {
|
|
if x != nil {
|
|
return x.TlsServerName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *CheckTLSConfig) GetTlsSkipVerify() bool {
|
|
if x != nil {
|
|
return x.TlsSkipVerify
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *CheckTLSConfig) GetUseTls() bool {
|
|
if x != nil {
|
|
return x.UseTls
|
|
}
|
|
return false
|
|
}
|
|
|
|
var File_pbcatalog_v1alpha1_health_proto protoreflect.FileDescriptor
|
|
|
|
var file_pbcatalog_v1alpha1_health_proto_rawDesc = []byte{
|
|
0x0a, 0x1f, 0x70, 0x62, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2f, 0x76, 0x31, 0x61, 0x6c,
|
|
0x70, 0x68, 0x61, 0x31, 0x2f, 0x68, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x12, 0x21, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e,
|
|
0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c,
|
|
0x70, 0x68, 0x61, 0x31, 0x1a, 0x1e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x64, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x2e, 0x70,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x21, 0x70, 0x62, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2f,
|
|
0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2f, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x6f,
|
|
0x72, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x22, 0x9f, 0x01, 0x0a, 0x0c, 0x48, 0x65, 0x61, 0x6c,
|
|
0x74, 0x68, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x12, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x12, 0x41, 0x0a, 0x06,
|
|
0x73, 0x74, 0x61, 0x74, 0x75, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x29, 0x2e, 0x68,
|
|
0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e,
|
|
0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31,
|
|
0x2e, 0x48, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x52, 0x06, 0x73, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12,
|
|
0x20, 0x0a, 0x0b, 0x64, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x64, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x12, 0x16, 0x0a, 0x06, 0x6f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x18, 0x04, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x06, 0x6f, 0x75, 0x74, 0x70, 0x75, 0x74, 0x22, 0xb6, 0x01, 0x0a, 0x0c, 0x48, 0x65,
|
|
0x61, 0x6c, 0x74, 0x68, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x73, 0x12, 0x51, 0x0a, 0x09, 0x77, 0x6f,
|
|
0x72, 0x6b, 0x6c, 0x6f, 0x61, 0x64, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x33, 0x2e,
|
|
0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c,
|
|
0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61,
|
|
0x31, 0x2e, 0x57, 0x6f, 0x72, 0x6b, 0x6c, 0x6f, 0x61, 0x64, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74,
|
|
0x6f, 0x72, 0x52, 0x09, 0x77, 0x6f, 0x72, 0x6b, 0x6c, 0x6f, 0x61, 0x64, 0x73, 0x12, 0x53, 0x0a,
|
|
0x0d, 0x68, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x5f, 0x63, 0x68, 0x65, 0x63, 0x6b, 0x73, 0x18, 0x02,
|
|
0x20, 0x03, 0x28, 0x0b, 0x32, 0x2e, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70,
|
|
0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e,
|
|
0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2e, 0x48, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x43,
|
|
0x68, 0x65, 0x63, 0x6b, 0x52, 0x0c, 0x68, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x43, 0x68, 0x65, 0x63,
|
|
0x6b, 0x73, 0x22, 0xd0, 0x04, 0x0a, 0x0b, 0x48, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x43, 0x68, 0x65,
|
|
0x63, 0x6b, 0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x12, 0x42, 0x0a, 0x04, 0x68, 0x74, 0x74, 0x70, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x2c, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70,
|
|
0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e,
|
|
0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2e, 0x48, 0x54, 0x54, 0x50, 0x43, 0x68, 0x65,
|
|
0x63, 0x6b, 0x48, 0x00, 0x52, 0x04, 0x68, 0x74, 0x74, 0x70, 0x12, 0x3f, 0x0a, 0x03, 0x74, 0x63,
|
|
0x70, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x2b, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63,
|
|
0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c,
|
|
0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2e, 0x54, 0x43, 0x50, 0x43,
|
|
0x68, 0x65, 0x63, 0x6b, 0x48, 0x00, 0x52, 0x03, 0x74, 0x63, 0x70, 0x12, 0x3f, 0x0a, 0x03, 0x75,
|
|
0x64, 0x70, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x2b, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69,
|
|
0x63, 0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61,
|
|
0x6c, 0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2e, 0x55, 0x44, 0x50,
|
|
0x43, 0x68, 0x65, 0x63, 0x6b, 0x48, 0x00, 0x52, 0x03, 0x75, 0x64, 0x70, 0x12, 0x42, 0x0a, 0x04,
|
|
0x67, 0x72, 0x70, 0x63, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x2c, 0x2e, 0x68, 0x61, 0x73,
|
|
0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61,
|
|
0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2e, 0x47,
|
|
0x52, 0x50, 0x43, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x48, 0x00, 0x52, 0x04, 0x67, 0x72, 0x70, 0x63,
|
|
0x12, 0x52, 0x0a, 0x0a, 0x6f, 0x73, 0x5f, 0x73, 0x65, 0x72, 0x76, 0x69, 0x63, 0x65, 0x18, 0x06,
|
|
0x20, 0x01, 0x28, 0x0b, 0x32, 0x31, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70,
|
|
0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e,
|
|
0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2e, 0x4f, 0x53, 0x53, 0x65, 0x72, 0x76, 0x69,
|
|
0x63, 0x65, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x48, 0x00, 0x52, 0x09, 0x6f, 0x73, 0x53, 0x65, 0x72,
|
|
0x76, 0x69, 0x63, 0x65, 0x12, 0x35, 0x0a, 0x08, 0x69, 0x6e, 0x74, 0x65, 0x72, 0x76, 0x61, 0x6c,
|
|
0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x44, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x52, 0x08, 0x69, 0x6e, 0x74, 0x65, 0x72, 0x76, 0x61, 0x6c, 0x12, 0x33, 0x0a, 0x07, 0x74,
|
|
0x69, 0x6d, 0x65, 0x6f, 0x75, 0x74, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x67,
|
|
0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x44,
|
|
0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x07, 0x74, 0x69, 0x6d, 0x65, 0x6f, 0x75, 0x74,
|
|
0x12, 0x55, 0x0a, 0x19, 0x64, 0x65, 0x72, 0x65, 0x67, 0x69, 0x73, 0x74, 0x65, 0x72, 0x5f, 0x63,
|
|
0x72, 0x69, 0x74, 0x69, 0x63, 0x61, 0x6c, 0x5f, 0x61, 0x66, 0x74, 0x65, 0x72, 0x18, 0x09, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x44, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x17,
|
|
0x64, 0x65, 0x72, 0x65, 0x67, 0x69, 0x73, 0x74, 0x65, 0x72, 0x43, 0x72, 0x69, 0x74, 0x69, 0x63,
|
|
0x61, 0x6c, 0x41, 0x66, 0x74, 0x65, 0x72, 0x42, 0x0c, 0x0a, 0x0a, 0x64, 0x65, 0x66, 0x69, 0x6e,
|
|
0x69, 0x74, 0x69, 0x6f, 0x6e, 0x22, 0xc8, 0x02, 0x0a, 0x09, 0x48, 0x54, 0x54, 0x50, 0x43, 0x68,
|
|
0x65, 0x63, 0x6b, 0x12, 0x10, 0x0a, 0x03, 0x75, 0x72, 0x6c, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x03, 0x75, 0x72, 0x6c, 0x12, 0x50, 0x0a, 0x06, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x18,
|
|
0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x38, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72,
|
|
0x70, 0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67,
|
|
0x2e, 0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x2e, 0x48, 0x54, 0x54, 0x50, 0x43, 0x68,
|
|
0x65, 0x63, 0x6b, 0x2e, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52,
|
|
0x06, 0x68, 0x65, 0x61, 0x64, 0x65, 0x72, 0x12, 0x16, 0x0a, 0x06, 0x6d, 0x65, 0x74, 0x68, 0x6f,
|
|
0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x6d, 0x65, 0x74, 0x68, 0x6f, 0x64, 0x12,
|
|
0x12, 0x0a, 0x04, 0x62, 0x6f, 0x64, 0x79, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x62,
|
|
0x6f, 0x64, 0x79, 0x12, 0x2b, 0x0a, 0x11, 0x64, 0x69, 0x73, 0x61, 0x62, 0x6c, 0x65, 0x5f, 0x72,
|
|
0x65, 0x64, 0x69, 0x72, 0x65, 0x63, 0x74, 0x73, 0x18, 0x05, 0x20, 0x01, 0x28, 0x08, 0x52, 0x10,
|
|
0x64, 0x69, 0x73, 0x61, 0x62, 0x6c, 0x65, 0x52, 0x65, 0x64, 0x69, 0x72, 0x65, 0x63, 0x74, 0x73,
|
|
0x12, 0x43, 0x0a, 0x03, 0x74, 0x6c, 0x73, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x31, 0x2e,
|
|
0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c,
|
|
0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61,
|
|
0x31, 0x2e, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x54, 0x4c, 0x53, 0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67,
|
|
0x52, 0x03, 0x74, 0x6c, 0x73, 0x1a, 0x39, 0x0a, 0x0b, 0x48, 0x65, 0x61, 0x64, 0x65, 0x72, 0x45,
|
|
0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01,
|
|
0x22, 0x24, 0x0a, 0x08, 0x54, 0x43, 0x50, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x12, 0x18, 0x0a, 0x07,
|
|
0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61,
|
|
0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x22, 0x24, 0x0a, 0x08, 0x55, 0x44, 0x50, 0x43, 0x68, 0x65,
|
|
0x63, 0x6b, 0x12, 0x18, 0x0a, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x22, 0x6a, 0x0a, 0x09,
|
|
0x47, 0x52, 0x50, 0x43, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x12, 0x18, 0x0a, 0x07, 0x61, 0x64, 0x64,
|
|
0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61, 0x64, 0x64, 0x72,
|
|
0x65, 0x73, 0x73, 0x12, 0x43, 0x0a, 0x03, 0x74, 0x6c, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x31, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2e, 0x63, 0x6f, 0x6e,
|
|
0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e, 0x76, 0x31, 0x61, 0x6c,
|
|
0x70, 0x68, 0x61, 0x31, 0x2e, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x54, 0x4c, 0x53, 0x43, 0x6f, 0x6e,
|
|
0x66, 0x69, 0x67, 0x52, 0x03, 0x74, 0x6c, 0x73, 0x22, 0x2a, 0x0a, 0x0e, 0x4f, 0x53, 0x53, 0x65,
|
|
0x72, 0x76, 0x69, 0x63, 0x65, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x12, 0x18, 0x0a, 0x07, 0x61, 0x64,
|
|
0x64, 0x72, 0x65, 0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x61, 0x64, 0x64,
|
|
0x72, 0x65, 0x73, 0x73, 0x22, 0x79, 0x0a, 0x0e, 0x43, 0x68, 0x65, 0x63, 0x6b, 0x54, 0x4c, 0x53,
|
|
0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x12, 0x26, 0x0a, 0x0f, 0x74, 0x6c, 0x73, 0x5f, 0x73, 0x65,
|
|
0x72, 0x76, 0x65, 0x72, 0x5f, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x0d, 0x74, 0x6c, 0x73, 0x53, 0x65, 0x72, 0x76, 0x65, 0x72, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x26,
|
|
0x0a, 0x0f, 0x74, 0x6c, 0x73, 0x5f, 0x73, 0x6b, 0x69, 0x70, 0x5f, 0x76, 0x65, 0x72, 0x69, 0x66,
|
|
0x79, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0d, 0x74, 0x6c, 0x73, 0x53, 0x6b, 0x69, 0x70,
|
|
0x56, 0x65, 0x72, 0x69, 0x66, 0x79, 0x12, 0x17, 0x0a, 0x07, 0x75, 0x73, 0x65, 0x5f, 0x74, 0x6c,
|
|
0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x06, 0x75, 0x73, 0x65, 0x54, 0x6c, 0x73, 0x2a,
|
|
0x6d, 0x0a, 0x06, 0x48, 0x65, 0x61, 0x6c, 0x74, 0x68, 0x12, 0x0e, 0x0a, 0x0a, 0x48, 0x45, 0x41,
|
|
0x4c, 0x54, 0x48, 0x5f, 0x41, 0x4e, 0x59, 0x10, 0x00, 0x12, 0x12, 0x0a, 0x0e, 0x48, 0x45, 0x41,
|
|
0x4c, 0x54, 0x48, 0x5f, 0x50, 0x41, 0x53, 0x53, 0x49, 0x4e, 0x47, 0x10, 0x01, 0x12, 0x12, 0x0a,
|
|
0x0e, 0x48, 0x45, 0x41, 0x4c, 0x54, 0x48, 0x5f, 0x57, 0x41, 0x52, 0x4e, 0x49, 0x4e, 0x47, 0x10,
|
|
0x02, 0x12, 0x13, 0x0a, 0x0f, 0x48, 0x45, 0x41, 0x4c, 0x54, 0x48, 0x5f, 0x43, 0x52, 0x49, 0x54,
|
|
0x49, 0x43, 0x41, 0x4c, 0x10, 0x03, 0x12, 0x16, 0x0a, 0x12, 0x48, 0x45, 0x41, 0x4c, 0x54, 0x48,
|
|
0x5f, 0x4d, 0x41, 0x49, 0x4e, 0x54, 0x45, 0x4e, 0x41, 0x4e, 0x43, 0x45, 0x10, 0x04, 0x42, 0xa8,
|
|
0x02, 0x0a, 0x25, 0x63, 0x6f, 0x6d, 0x2e, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70,
|
|
0x2e, 0x63, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e,
|
|
0x76, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x42, 0x0b, 0x48, 0x65, 0x61, 0x6c, 0x74, 0x68,
|
|
0x50, 0x72, 0x6f, 0x74, 0x6f, 0x50, 0x01, 0x5a, 0x4b, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e,
|
|
0x63, 0x6f, 0x6d, 0x2f, 0x68, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2f, 0x63, 0x6f,
|
|
0x6e, 0x73, 0x75, 0x6c, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x2d, 0x70, 0x75, 0x62, 0x6c, 0x69,
|
|
0x63, 0x2f, 0x70, 0x62, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2f, 0x76, 0x31, 0x61, 0x6c,
|
|
0x70, 0x68, 0x61, 0x31, 0x3b, 0x63, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x76, 0x31, 0x61, 0x6c,
|
|
0x70, 0x68, 0x61, 0x31, 0xa2, 0x02, 0x03, 0x48, 0x43, 0x43, 0xaa, 0x02, 0x21, 0x48, 0x61, 0x73,
|
|
0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x2e, 0x43, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x2e, 0x43, 0x61,
|
|
0x74, 0x61, 0x6c, 0x6f, 0x67, 0x2e, 0x56, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0xca, 0x02,
|
|
0x21, 0x48, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x5c, 0x43, 0x6f, 0x6e, 0x73, 0x75,
|
|
0x6c, 0x5c, 0x43, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x5c, 0x56, 0x31, 0x61, 0x6c, 0x70, 0x68,
|
|
0x61, 0x31, 0xe2, 0x02, 0x2d, 0x48, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x5c, 0x43,
|
|
0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x5c, 0x43, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x5c, 0x56, 0x31,
|
|
0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x5c, 0x47, 0x50, 0x42, 0x4d, 0x65, 0x74, 0x61, 0x64, 0x61,
|
|
0x74, 0x61, 0xea, 0x02, 0x24, 0x48, 0x61, 0x73, 0x68, 0x69, 0x63, 0x6f, 0x72, 0x70, 0x3a, 0x3a,
|
|
0x43, 0x6f, 0x6e, 0x73, 0x75, 0x6c, 0x3a, 0x3a, 0x43, 0x61, 0x74, 0x61, 0x6c, 0x6f, 0x67, 0x3a,
|
|
0x3a, 0x56, 0x31, 0x61, 0x6c, 0x70, 0x68, 0x61, 0x31, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x33,
|
|
}
|
|
|
|
var (
|
|
file_pbcatalog_v1alpha1_health_proto_rawDescOnce sync.Once
|
|
file_pbcatalog_v1alpha1_health_proto_rawDescData = file_pbcatalog_v1alpha1_health_proto_rawDesc
|
|
)
|
|
|
|
func file_pbcatalog_v1alpha1_health_proto_rawDescGZIP() []byte {
|
|
file_pbcatalog_v1alpha1_health_proto_rawDescOnce.Do(func() {
|
|
file_pbcatalog_v1alpha1_health_proto_rawDescData = protoimpl.X.CompressGZIP(file_pbcatalog_v1alpha1_health_proto_rawDescData)
|
|
})
|
|
return file_pbcatalog_v1alpha1_health_proto_rawDescData
|
|
}
|
|
|
|
var file_pbcatalog_v1alpha1_health_proto_enumTypes = make([]protoimpl.EnumInfo, 1)
|
|
var file_pbcatalog_v1alpha1_health_proto_msgTypes = make([]protoimpl.MessageInfo, 10)
|
|
var file_pbcatalog_v1alpha1_health_proto_goTypes = []interface{}{
|
|
(Health)(0), // 0: hashicorp.consul.catalog.v1alpha1.Health
|
|
(*HealthStatus)(nil), // 1: hashicorp.consul.catalog.v1alpha1.HealthStatus
|
|
(*HealthChecks)(nil), // 2: hashicorp.consul.catalog.v1alpha1.HealthChecks
|
|
(*HealthCheck)(nil), // 3: hashicorp.consul.catalog.v1alpha1.HealthCheck
|
|
(*HTTPCheck)(nil), // 4: hashicorp.consul.catalog.v1alpha1.HTTPCheck
|
|
(*TCPCheck)(nil), // 5: hashicorp.consul.catalog.v1alpha1.TCPCheck
|
|
(*UDPCheck)(nil), // 6: hashicorp.consul.catalog.v1alpha1.UDPCheck
|
|
(*GRPCCheck)(nil), // 7: hashicorp.consul.catalog.v1alpha1.GRPCCheck
|
|
(*OSServiceCheck)(nil), // 8: hashicorp.consul.catalog.v1alpha1.OSServiceCheck
|
|
(*CheckTLSConfig)(nil), // 9: hashicorp.consul.catalog.v1alpha1.CheckTLSConfig
|
|
nil, // 10: hashicorp.consul.catalog.v1alpha1.HTTPCheck.HeaderEntry
|
|
(*WorkloadSelector)(nil), // 11: hashicorp.consul.catalog.v1alpha1.WorkloadSelector
|
|
(*durationpb.Duration)(nil), // 12: google.protobuf.Duration
|
|
}
|
|
var file_pbcatalog_v1alpha1_health_proto_depIdxs = []int32{
|
|
0, // 0: hashicorp.consul.catalog.v1alpha1.HealthStatus.status:type_name -> hashicorp.consul.catalog.v1alpha1.Health
|
|
11, // 1: hashicorp.consul.catalog.v1alpha1.HealthChecks.workloads:type_name -> hashicorp.consul.catalog.v1alpha1.WorkloadSelector
|
|
3, // 2: hashicorp.consul.catalog.v1alpha1.HealthChecks.health_checks:type_name -> hashicorp.consul.catalog.v1alpha1.HealthCheck
|
|
4, // 3: hashicorp.consul.catalog.v1alpha1.HealthCheck.http:type_name -> hashicorp.consul.catalog.v1alpha1.HTTPCheck
|
|
5, // 4: hashicorp.consul.catalog.v1alpha1.HealthCheck.tcp:type_name -> hashicorp.consul.catalog.v1alpha1.TCPCheck
|
|
6, // 5: hashicorp.consul.catalog.v1alpha1.HealthCheck.udp:type_name -> hashicorp.consul.catalog.v1alpha1.UDPCheck
|
|
7, // 6: hashicorp.consul.catalog.v1alpha1.HealthCheck.grpc:type_name -> hashicorp.consul.catalog.v1alpha1.GRPCCheck
|
|
8, // 7: hashicorp.consul.catalog.v1alpha1.HealthCheck.os_service:type_name -> hashicorp.consul.catalog.v1alpha1.OSServiceCheck
|
|
12, // 8: hashicorp.consul.catalog.v1alpha1.HealthCheck.interval:type_name -> google.protobuf.Duration
|
|
12, // 9: hashicorp.consul.catalog.v1alpha1.HealthCheck.timeout:type_name -> google.protobuf.Duration
|
|
12, // 10: hashicorp.consul.catalog.v1alpha1.HealthCheck.deregister_critical_after:type_name -> google.protobuf.Duration
|
|
10, // 11: hashicorp.consul.catalog.v1alpha1.HTTPCheck.header:type_name -> hashicorp.consul.catalog.v1alpha1.HTTPCheck.HeaderEntry
|
|
9, // 12: hashicorp.consul.catalog.v1alpha1.HTTPCheck.tls:type_name -> hashicorp.consul.catalog.v1alpha1.CheckTLSConfig
|
|
9, // 13: hashicorp.consul.catalog.v1alpha1.GRPCCheck.tls:type_name -> hashicorp.consul.catalog.v1alpha1.CheckTLSConfig
|
|
14, // [14:14] is the sub-list for method output_type
|
|
14, // [14:14] is the sub-list for method input_type
|
|
14, // [14:14] is the sub-list for extension type_name
|
|
14, // [14:14] is the sub-list for extension extendee
|
|
0, // [0:14] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_pbcatalog_v1alpha1_health_proto_init() }
|
|
func file_pbcatalog_v1alpha1_health_proto_init() {
|
|
if File_pbcatalog_v1alpha1_health_proto != nil {
|
|
return
|
|
}
|
|
file_pbcatalog_v1alpha1_selector_proto_init()
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*HealthStatus); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*HealthChecks); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*HealthCheck); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*HTTPCheck); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*TCPCheck); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UDPCheck); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*GRPCCheck); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*OSServiceCheck); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*CheckTLSConfig); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
file_pbcatalog_v1alpha1_health_proto_msgTypes[2].OneofWrappers = []interface{}{
|
|
(*HealthCheck_Http)(nil),
|
|
(*HealthCheck_Tcp)(nil),
|
|
(*HealthCheck_Udp)(nil),
|
|
(*HealthCheck_Grpc)(nil),
|
|
(*HealthCheck_OsService)(nil),
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_pbcatalog_v1alpha1_health_proto_rawDesc,
|
|
NumEnums: 1,
|
|
NumMessages: 10,
|
|
NumExtensions: 0,
|
|
NumServices: 0,
|
|
},
|
|
GoTypes: file_pbcatalog_v1alpha1_health_proto_goTypes,
|
|
DependencyIndexes: file_pbcatalog_v1alpha1_health_proto_depIdxs,
|
|
EnumInfos: file_pbcatalog_v1alpha1_health_proto_enumTypes,
|
|
MessageInfos: file_pbcatalog_v1alpha1_health_proto_msgTypes,
|
|
}.Build()
|
|
File_pbcatalog_v1alpha1_health_proto = out.File
|
|
file_pbcatalog_v1alpha1_health_proto_rawDesc = nil
|
|
file_pbcatalog_v1alpha1_health_proto_goTypes = nil
|
|
file_pbcatalog_v1alpha1_health_proto_depIdxs = nil
|
|
}
|