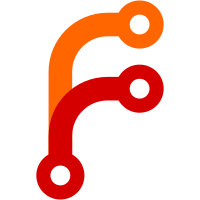
This commit adds 2 ember component/helpers and a service to contain the shared functionality for matching/rendering content dependent on state identifiers. Currently a `service.state` method has been added to easily make manual state objects, but these are built towards using `xstate` to manage UI state in some of our future components. We've added some tests here, and we aren't currently using these components anywhere in this commit.
28 lines
1.2 KiB
JavaScript
28 lines
1.2 KiB
JavaScript
import { module, test } from 'qunit';
|
|
import { setupTest } from 'ember-qunit';
|
|
|
|
module('Unit | Service | state', function(hooks) {
|
|
setupTest(hooks);
|
|
|
|
// Replace this with your real tests.
|
|
test('.state creates a state matchable object', function(assert) {
|
|
const service = this.owner.lookup('service:state');
|
|
const actual = service.state(id => id === 'idle');
|
|
assert.equal(typeof actual, 'object');
|
|
assert.equal(typeof actual.matches, 'function');
|
|
});
|
|
test('.matches performs a match correctly', function(assert) {
|
|
const service = this.owner.lookup('service:state');
|
|
const state = service.state(id => id === 'idle');
|
|
assert.equal(service.matches(state, 'idle'), true);
|
|
assert.equal(service.matches(state, 'loading'), false);
|
|
});
|
|
test('.matches performs a match correctly when passed an array', function(assert) {
|
|
const service = this.owner.lookup('service:state');
|
|
const state = service.state(id => id === 'idle');
|
|
assert.equal(service.matches(state, ['idle']), true);
|
|
assert.equal(service.matches(state, ['loading', 'idle']), true);
|
|
assert.equal(service.matches(state, ['loading', 'deleting']), false);
|
|
});
|
|
});
|