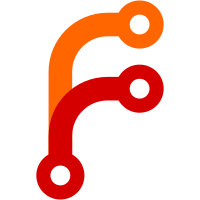
This is only configured in xDS when a service with an L7 protocol is exported. They also load any relevant trust bundles for the peered services to eventually use for L7 SPIFFE validation during mTLS termination.
31 lines
632 B
Go
31 lines
632 B
Go
package connect
|
|
|
|
import (
|
|
"net/url"
|
|
|
|
"github.com/hashicorp/consul/acl"
|
|
)
|
|
|
|
type SpiffeIDMeshGateway struct {
|
|
Host string
|
|
Partition string
|
|
Datacenter string
|
|
}
|
|
|
|
func (id SpiffeIDMeshGateway) MatchesPartition(partition string) bool {
|
|
return id.PartitionOrDefault() == acl.PartitionOrDefault(partition)
|
|
}
|
|
|
|
func (id SpiffeIDMeshGateway) PartitionOrDefault() string {
|
|
return acl.PartitionOrDefault(id.Partition)
|
|
}
|
|
|
|
// URI returns the *url.URL for this SPIFFE ID.
|
|
func (id SpiffeIDMeshGateway) URI() *url.URL {
|
|
var result url.URL
|
|
result.Scheme = "spiffe"
|
|
result.Host = id.Host
|
|
result.Path = id.uriPath()
|
|
return &result
|
|
}
|