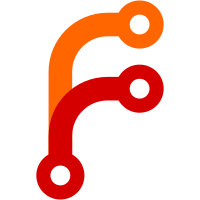
The Consul API can pass through `Value: null` which does not get cast to a string by ember-data. This snowballs into problems with `atob` which then tried to decode `null`. There are 2 problems here. 1. `Value` should never be `null` - I've added a removeNull function to shallowly loop though props and remove properties that are `null`, for the moment this is only on single KV JSON responses - therefore `Value` will never be `null` which is the root of the problem 2. `atob` doesn't quite follow the `window.atob` API in that the `window.atob` API casts everything down to a string first, therefore it will try to decode `null` > `'null'` > `crazy unicode thing`. - I've commented in a fix for this, but whilst this shouldn't be causing anymore problems in our UI (now that `Value` is never `null`), I'll uncomment it in another future release. Tests are already written for it which more closely follow `window.atob` but skipped for now (next commit)
54 lines
1.7 KiB
JavaScript
54 lines
1.7 KiB
JavaScript
/**
|
|
* super stubber
|
|
* Ember's `_super` functionality is a little challenging to stub.
|
|
* The following will essentially let you stub `_super`, letting
|
|
* you test single units of code that use `_super`
|
|
* The return value is a function with the same signature as
|
|
* traditional `test` or `it` test functions. Any test code
|
|
* used within the cb 'sandbox' will use the stubbed `_super`.
|
|
* It's done this way to attempt to make it easy to reuse for various tests
|
|
* using the same stub in a recognisable way.
|
|
*
|
|
* @param {object} obj - The instance with the that `_super` belongs to
|
|
* @param {object} [stub=function(){return arguments}] -
|
|
* The stub to use in place of `_super`, if not specified `_super` will
|
|
* simply return the `arguments` passed to it
|
|
*
|
|
* @returns {function}
|
|
*/
|
|
export default function(obj, stub) {
|
|
const _super =
|
|
typeof stub === 'undefined'
|
|
? function() {
|
|
return arguments;
|
|
}
|
|
: stub;
|
|
/**
|
|
* @param {string} message - Message to accompany the test concept (currently unused)
|
|
* @param {function} cb - Callback that performs the test, will use the stubbed `_super`
|
|
* @returns The result of `cb`, and therefore maintain the same API
|
|
*/
|
|
return function(message, _cb) {
|
|
const cb = typeof message === 'function' ? message : _cb;
|
|
|
|
let orig = obj._super;
|
|
Object.defineProperty(Object.getPrototypeOf(obj), '_super', {
|
|
set: function() {},
|
|
get: function() {
|
|
return _super;
|
|
},
|
|
});
|
|
// TODO: try/catch this?
|
|
const actual = cb();
|
|
Object.defineProperty(Object.getPrototypeOf(obj), '_super', {
|
|
set: function(val) {
|
|
orig = val;
|
|
},
|
|
get: function() {
|
|
return orig;
|
|
},
|
|
});
|
|
return actual;
|
|
};
|
|
}
|