mirror of
https://github.com/facebook/rocksdb.git
synced 2024-11-25 22:44:05 +00:00
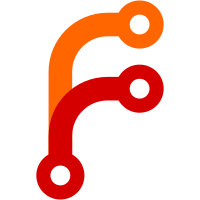
Summary: This PR implements a new admission policy for the compressed secondary cache, which includes the functionality of the existing policy, and also admits items evicted from the primary block cache with the hit bit set. Effectively, the new policy works as follows - 1. When an item is demoted from the primary cache without a hit, a placeholder is inserted in the compressed cache. A second demotion will insert the full entry. 2. When an item is promoted from the compressed cache to the primary cache for the first time, a placeholder is inserted in the primary. The second promotion inserts the full entry, while erasing it form the compressed cache. 3. If an item is demoted from the primary cache with the hit bit set, it is immediately inserted in the compressed secondary cache. The ```TieredVolatileCacheOptions``` has been updated with a new option, ```adm_policy```, which allows the policy to be selected. Pull Request resolved: https://github.com/facebook/rocksdb/pull/11713 Reviewed By: pdillinger Differential Revision: D48444512 Pulled By: anand1976 fbshipit-source-id: b4cbf8c169a88097dff08e36e8bc4b3088de1492
46 lines
1.5 KiB
C++
46 lines
1.5 KiB
C++
// Copyright (c) 2011-present, Facebook, Inc. All rights reserved.
|
|
// This source code is licensed under both the GPLv2 (found in the
|
|
// COPYING file in the root directory) and Apache 2.0 License
|
|
// (found in the LICENSE.Apache file in the root directory).
|
|
|
|
#include "rocksdb/secondary_cache.h"
|
|
|
|
#include "cache/cache_entry_roles.h"
|
|
|
|
namespace ROCKSDB_NAMESPACE {
|
|
|
|
namespace {
|
|
|
|
void NoopDelete(Cache::ObjectPtr, MemoryAllocator*) {}
|
|
|
|
size_t SliceSize(Cache::ObjectPtr obj) {
|
|
return static_cast<Slice*>(obj)->size();
|
|
}
|
|
|
|
Status SliceSaveTo(Cache::ObjectPtr from_obj, size_t from_offset, size_t length,
|
|
char* out) {
|
|
const Slice& slice = *static_cast<Slice*>(from_obj);
|
|
std::memcpy(out, slice.data() + from_offset, length);
|
|
return Status::OK();
|
|
}
|
|
|
|
Status FailCreate(const Slice&, Cache::CreateContext*, MemoryAllocator*,
|
|
Cache::ObjectPtr*, size_t*) {
|
|
return Status::NotSupported("Only for dumping data into SecondaryCache");
|
|
}
|
|
|
|
} // namespace
|
|
|
|
Status SecondaryCache::InsertSaved(const Slice& key, const Slice& saved) {
|
|
static Cache::CacheItemHelper helper_no_secondary{CacheEntryRole::kMisc,
|
|
&NoopDelete};
|
|
static Cache::CacheItemHelper helper{
|
|
CacheEntryRole::kMisc, &NoopDelete, &SliceSize,
|
|
&SliceSaveTo, &FailCreate, &helper_no_secondary};
|
|
// NOTE: depends on Insert() being synchronous, not keeping pointer `&saved`
|
|
return Insert(key, const_cast<Slice*>(&saved), &helper,
|
|
/*force_insert=*/true);
|
|
}
|
|
|
|
} // namespace ROCKSDB_NAMESPACE
|