mirror of
https://github.com/facebook/rocksdb.git
synced 2024-11-26 16:30:56 +00:00
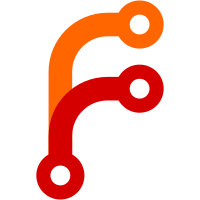
Summary: Right now UserComparatorWrapper is a Customizable object, although it is not, which introduces some intialization overhead for the object. In some benchmarks, it shows up in CPU profiling. Make it not configurable by defining most functions needed by UserComparatorWrapper to an interface and implement the interface. Pull Request resolved: https://github.com/facebook/rocksdb/pull/10837 Test Plan: Make sure existing tests pass Reviewed By: pdillinger Differential Revision: D40528511 fbshipit-source-id: 70eaac89ecd55401a26e8ed32abbc413a9617c62
65 lines
2.2 KiB
C++
65 lines
2.2 KiB
C++
// Copyright (c) 2011-present, Facebook, Inc. All rights reserved.
|
|
// This source code is licensed under both the GPLv2 (found in the
|
|
// COPYING file in the root directory) and Apache 2.0 License
|
|
// (found in the LICENSE.Apache file in the root directory).
|
|
// Copyright (c) 2011 The LevelDB Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file. See the AUTHORS file for names of contributors.
|
|
|
|
#pragma once
|
|
|
|
#include "monitoring/perf_context_imp.h"
|
|
#include "rocksdb/comparator.h"
|
|
|
|
namespace ROCKSDB_NAMESPACE {
|
|
|
|
// Wrapper of user comparator, with auto increment to
|
|
// perf_context.user_key_comparison_count.
|
|
class UserComparatorWrapper {
|
|
public:
|
|
// `UserComparatorWrapper`s constructed with the default constructor are not
|
|
// usable and will segfault on any attempt to use them for comparisons.
|
|
UserComparatorWrapper() : user_comparator_(nullptr) {}
|
|
|
|
explicit UserComparatorWrapper(const Comparator* const user_cmp)
|
|
: user_comparator_(user_cmp) {}
|
|
|
|
~UserComparatorWrapper() = default;
|
|
|
|
const Comparator* user_comparator() const { return user_comparator_; }
|
|
|
|
int Compare(const Slice& a, const Slice& b) const {
|
|
PERF_COUNTER_ADD(user_key_comparison_count, 1);
|
|
return user_comparator_->Compare(a, b);
|
|
}
|
|
|
|
bool Equal(const Slice& a, const Slice& b) const {
|
|
PERF_COUNTER_ADD(user_key_comparison_count, 1);
|
|
return user_comparator_->Equal(a, b);
|
|
}
|
|
|
|
int CompareTimestamp(const Slice& ts1, const Slice& ts2) const {
|
|
return user_comparator_->CompareTimestamp(ts1, ts2);
|
|
}
|
|
|
|
int CompareWithoutTimestamp(const Slice& a, const Slice& b) const {
|
|
PERF_COUNTER_ADD(user_key_comparison_count, 1);
|
|
return user_comparator_->CompareWithoutTimestamp(a, b);
|
|
}
|
|
|
|
int CompareWithoutTimestamp(const Slice& a, bool a_has_ts, const Slice& b,
|
|
bool b_has_ts) const {
|
|
PERF_COUNTER_ADD(user_key_comparison_count, 1);
|
|
return user_comparator_->CompareWithoutTimestamp(a, a_has_ts, b, b_has_ts);
|
|
}
|
|
|
|
bool EqualWithoutTimestamp(const Slice& a, const Slice& b) const {
|
|
return user_comparator_->EqualWithoutTimestamp(a, b);
|
|
}
|
|
|
|
private:
|
|
const Comparator* user_comparator_;
|
|
};
|
|
|
|
} // namespace ROCKSDB_NAMESPACE
|