mirror of
https://github.com/bazel-contrib/rules_foreign_cc
synced 2024-12-04 08:02:31 +00:00
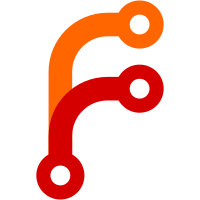
this way the users can influence which install prefix will appear in the generated code install prefix have to be relative - we are doing the hermetic build modify our shell script to copy the result of the build into the target directory (we can not leave it in temp directory, it will be deleted)
121 lines
2.9 KiB
Bash
121 lines
2.9 KiB
Bash
#!/usr/bin/env bash
|
|
|
|
# default placeholder value
|
|
REPLACE_VALUE='BAZEL_GEN_ROOT'
|
|
|
|
# Echo variables in the format
|
|
# var1_name="var1_value"
|
|
# ...
|
|
# vark_name="vark_value"
|
|
#
|
|
# arguments: the names of the variables
|
|
function echo_vars() {
|
|
for arg in "$@"
|
|
do
|
|
echo_var "$arg"
|
|
done
|
|
}
|
|
|
|
# Echo variable in the format var_name="var_value"
|
|
# $1 the name of the variable
|
|
function echo_var() {
|
|
local name="$1"
|
|
local value=${!name}
|
|
echo "$name: \"${value}\""
|
|
}
|
|
|
|
# Wrap the function execution in the echo lines:
|
|
# --- START $1
|
|
# (anything printed by $2)
|
|
# --- END $1
|
|
# $1 parameter to be printed next to start/end text
|
|
# $2 function to call in between START and END
|
|
function wrap() {
|
|
echo "--- START $1:"
|
|
$2
|
|
echo "--- END $1"
|
|
}
|
|
|
|
# Append string to PATH variable
|
|
# $1 string to append
|
|
function path() {
|
|
export PATH="$1:$PATH"
|
|
}
|
|
|
|
# Replace string in all files in directory
|
|
# $1 directory to search recursively, absolute path
|
|
# $2 string to replace
|
|
# $3 replace target
|
|
function replace_in_files() {
|
|
if [ -d "$1" ]; then
|
|
find $1 -type f,l -exec sed -i 's@'"$2"'@'"$3"'@g' {} ';'
|
|
fi
|
|
}
|
|
|
|
# copies contents of the directory to target directory (create the target directory if needed)
|
|
# $1 source directory, immediate children of which are copied
|
|
# $2 target directory
|
|
function copy_dir_contents_to_dir() {
|
|
local children=$(find $1 -maxdepth 1)
|
|
local target="$2"
|
|
mkdir -p ${target}
|
|
for child in $children; do
|
|
cp -R $child ${target}
|
|
done
|
|
}
|
|
|
|
# Symlink contents of the directory to target directory (create the target directory if needed)
|
|
# $1 source directory, immediate children of which are symlinked
|
|
# $2 target directory
|
|
function symlink_dir_contents_to_dir() {
|
|
local children=$(find $1 -maxdepth 1)
|
|
local target="$2"
|
|
mkdir -p ${target}
|
|
for child in $children; do
|
|
symlink_to_dir $child ${target}
|
|
done
|
|
}
|
|
|
|
# Symlink all files from source directory to target directory (create the target directory if needed)
|
|
# NB symlinks from the source directory are copied
|
|
# $1 source directory
|
|
# $2 target directory
|
|
function symlink_to_dir() {
|
|
local target="$2"
|
|
mkdir -p ${target}
|
|
|
|
if [[ -d $1 ]]; then
|
|
ln -s -t ${target} $1
|
|
elif [[ -f $1 ]]; then
|
|
ln -s -t ${target} $1
|
|
elif [[ -L $1 ]]; then
|
|
cp $1 ${target}
|
|
else
|
|
echo "Can not copy $1"
|
|
fi
|
|
}
|
|
|
|
# Copy all files from source directory to target directory (create the target directory if needed),
|
|
# and add target paths on to path
|
|
# $1 source directory
|
|
# $2 target directory
|
|
function copy_and_add_to_path() {
|
|
copy_to_dir $1 $2
|
|
path $2/bin
|
|
path $2
|
|
}
|
|
|
|
# replace placeholder with absolute path in all files in a directory
|
|
# $1 directory
|
|
# $2 absolute path
|
|
function define_absolute_paths() {
|
|
replace_in_files $1 $REPLACE_VALUE $2
|
|
}
|
|
|
|
# replace the absolute path with a placeholder value in all files in a directory
|
|
# $1 directory
|
|
# $2 absolute path to replace
|
|
function replace_absolute_paths() {
|
|
replace_in_files $1 $2 $REPLACE_VALUE
|
|
}
|